C++ Programming for Beginners (2023) - Video Tutorials
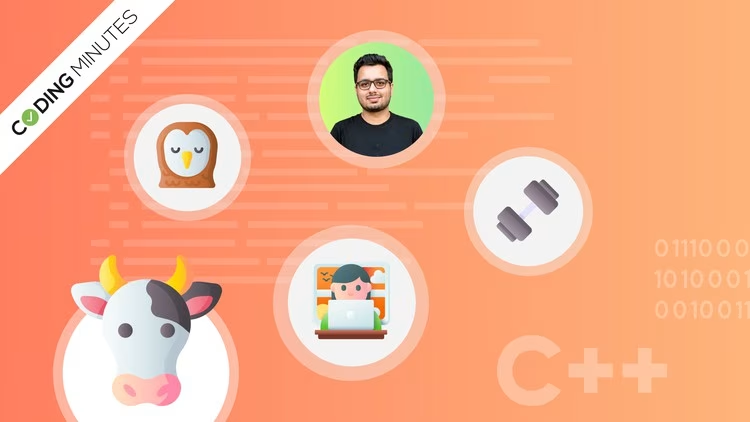
- 19h 37m Duration
- 199 Lectures
- 6921 Views
C++ Programming for Beginners (2023) - Video Tutorials
Discover our comprehensive C++ Programming for Beginners course, featuring over 30+ hours of engaging video content. This immersive educational experience covers essential programming fundamentals, from variables and data types to advanced concepts like object-oriented programming, memory management, and file handling. Our active and interactive teaching approach ensures you grasp every concept with clarity and confidence.
Learning Outcomes:
- Master Programming Fundamentals & Concepts
- Translate Logic into C++ Code Proficiently
- Gain Expertise in Modern C++ Syntax
- Explore the World of Object-Oriented Programming
- Harness the Power of the C++ Standard Template Library
- Enhance Your Problem-Solving Skills
1. Course Introduction
- Introduction to Course
- Course Logistics
2. Logical Thinking-I Flowcharts
- Building Blocks of Flowchart
- Flowchart – Simple Interest
- Flowchart – Largest Number
- Flowchart – Sum of First N Numbers
- Flowchart – Sum of Multiple Inputs
- Flowchart – Prime Number
- Flowchart – GCD
- Flowchart – Bank Employee
- Flowchart – Bank Guard
- Assignment – Flowcharts
3. Logical Thinking-II Pseudocode
- Pseudocode – Notation
- Pseudocode – Slmple Interest
- Pseudocode – Sum 1 to N
- Pseudocode – Sum of N Numbers
- Pseudocode – Prime or Not
- Pseudocode – GCD
- Pseudocode – Star Pattern
- Pseudocode – Star Pyramid
- Pseducode Assignment
4. C++ Getting Started
- C-H- Boilerplate
- Sublime Text + Compiler Setup
- Building & Running Code
- Hello, World!
- Solution : Hello, World!
- Input & Output
- Square of Number
- Solution : Square of Number
5. C++ Diving Deeper
- Preprocessor Directive
- Identifiers
- Keywords
- Main C++ Programming for Beginners
- Namespaces
- Comments
- Summary
- Hello You!
- Solution – Hello You!
6. Variables, Datatypes & Storage
- Variables
- Datatypes
- Using sizeOf Operator
- Binary Number System
- Storage of Integers
- Data type Modifiers
- Storage of Negative Numbers
- Range of Signed vs Unsigned Integers
- Storage of Floats & Doubles
- Storage of Characters
- Storage of Booleans
- Constants
- Typecasting
- Explicit Typecasting
- Typecasting Challenge
- Find the sum
- Solution – Find the sum
- Find the Average Marks
- Solution – Find the Average Marks
- Find the Ceil
- Ceil, Floor & Round Functions
- Find the Floor
- Simple Interest Calculator
- Solution – Simple Interest Calculator
7. Operators & Expressions C++ Programming For Beginners
- Arithmetic Operators
- Assignment Operators
- Increment Decrement Operators
- Relational Operators
- Logical Operators
- Bitwise Operators – I
- Bitwise Operators – II
8. Flow Control – Conditional Statements
- If Statement
- If-Else
- If Else-if Else
- Multiple If Blocks
- Problem – Electricity Bill Calculator
- Ternary Operator
- Switch Case
- Problem – Calculator
- Odd or Even Number
- Cash Withdrawal
- Predict the Grade
- Electricity Bill Calculator
- Fixed Deposit Interest
- Simple Calculator
9. Flow Control – Loops
- While Loop
- While Example – Sum of N Inputs
- For Loop
- For – While Equivalence
- Problem – Sum of Digits of a Number
- Problem – Star Pattern
- Problem
- For ABCD Pattern
- Problem – Print Reverse of a Number
- Problem – Reverse the Actual Number
- Break
- Continue
- Problem – Prime Number Check
- Find Largest
- Do While
- Nested Loops
- Problem – Number Pyramid
- Loops
- Sum of digits
- Sum of odd digits
- Sum of digits at odd position
- Fizz Buzz Problem
- Check Prime Number
- Armstrong Number
- Factorial of a Number
- Fibonacci Series
- Prime Numbers Till
10. Pattern Problems
- Pattern 1
- Pattern 2
- Pattern 3
- Pattern 4
- Pattern 6
- Pattern 7
- Pattern 8
11. Functions
- Introduction to Functions
- Functions Code Demo
- Default Parameters
- Forward Declaration
- Problem – Find Factorial
- Problem – Find Binomial Coefficient
- Call Stack
- Call Stack Example
- Function Overloading
- Overloading using variable parameters
- Overloading – using different datatypes
- Problem – Check Prime (Linear)
- Problem – Check Prime (Sqrt N Optimisation)
- Prime Number Optimised Code
- Problem – Primes in Range
- Practice – Call Stack Analysis
- Problem – Binary to Decimal
- Problem – Decimal to Binary
- Sum of Digits
- Binary To Decimal
- Check Prime Number
- Find the Factorial of a given Number N.
- Power function.
- Greatest common divisor.
- Prime numbers.
- Find nCr.
- Fibonacci Number
- Trailing Zeroes in N
- Trailing Zeroes in N!
- Functions Quiz
12. Pointers
- Address Of Operator
- Pointer Variables
- Dereference Operator
- Pass by References – using Pointers
- Reference Variables
- Pass by Reference – Reference Variables
- Pointers
13. Arrays
- Arrays Introduction
- Array Creation
- Array Input
- Passing Arrays to Functions
- Print Reverse on Array
- Reverse an Array – Two Pointer
- Print all Subarrays
- Sorting Basics
- Sorting using Comparator
- find Missing number.
- Largest and Smallest Number.
- Median of sorted Array.
- Kth smallest element in the array.
- Move all negative elements to one side of the array
- Find intersection of the two arrays
- Find the frequency of the given element
- Pair Sum
- Three Sum
- Rotate an array by K
- Arrays Quiz
14. Sorting & Searching Basics
- Linear Search
- Binary Search
- Binary Search Code
- Searching
- Find the element in the array.
- Frequency of largest element.
- Count of one.
- Square root
- Bubble Sort
- Bubble Sort Code
- Selection Sort
- Selection Sort Code
- Insertion Sort
- Insertion Son Code
- Count of one in an array.
- Sort an array !
- Search element in sorted array
- Find first and last occurrence of an element in a sorted array
- Find the total number of occurrence of an element in an array
- Soft an array of O, 1 2
- Sum of each row and column
15. 2D Arrays
- 2D Array
- 2D Array Storage
- Wave Print
- Spiral Print
- Staircase Search
- Staircase Search Code
- Number of zeros.
- Check whether product of elements are positive or negative.
- Sum of even numbers.
- Sum of all diagonal elements
- Search a 2D Matrix
- Mirror Image
- Spiral Print
16. Pointers Vs Arrays
- Pointers vs Arrays
- Pointer Airthmetic
- Pointer to an Array
- Dereferencing Pointers to an Array
- 2D Arrays & Pointers
- 2D Arrays & Pointers – Deep Dive
17. Character Data Type
- Introduction
- ASCII Encoding
- Input using cin.get()
- ‘cctype’ Header
- Challenge – Shortest Path
- Solution – Shortest Path
- Challenge – ABCD Pattern
- Guess the Output
18. Character Arrays/ C-Style Strings
- Character Array Basics
- Inputing Char Arrays – cin.getline()
- Problem – Create ReadLine function
- Problem – Finding Length
- Library Functions in
- Challenge – Largest String
- Challenge – Password Checker
- String Comparison
- String Methods Demo
- Challenge – Count Letter Types
- 2D Character Arrays
- Largest character.
- Largest frequency character
- Is character array sorted ?
- Count of unique characters
- Rotate the matrix.
19. C++ Strings
- [Pre-requisite]
- Strings Introduction
- Strings Initialisation
- Input using getline()
- String Operators
- String Traversal
- Looking up String Methods – Documentation
- Find Method
- Challenge – Uncomment String
- Valid Anagram
- Is String a palindrome?
- Upper to Lower
- Largest Frequency character
- All Alphabets present or not ?
- Given string can be formed or not ?
- Sum of all elements below main diagonal.
- Roman to Integer
- Number of words
- Longest Common Prefix
- Remove All Adjacent Duplicates
- Check for Subsequence
- Binary String to Decimal
20. Dynamic Memory Allocation
- Dynamic Memory Allocation Introduction
- Dynamic Memory Allocation – ID Array
- Dynamic Memory Allocation – 2D Array
- Dynamic Memory Allocation
21. Vectors
- Vectors Introduction
- Vectors – Behind the Scenes
- Vector Demo
- 2D Vectors
- Sort a vector of integers
- Merge two vectors
- Sort a vector of strings
- Maximum element
- Set Matrix Zeroes
22. Bitmasking Techniques
- Check Odd/Even Number
- Get ith Bit
- Set ith Bit
- Clear ith Bit
- Update ith Bit
- Clear last i bits
- Clear Bits in Range
- Challenge – Replace Bits
- Challenge – Power of 2
- Counting Set Bits
- Fast Exponentiation
- Decimal to Binary
- Count of set bit.
- Power of two.
- Divide a integer without Division operator
- Find square of a number without multiplication or divisor.
- Find a number which is singly present.
- Bitmasking
23. Recursion Introduction
- Recursion Introduction
- Factorial
- Fibonacci
- Sorted Array
- Increasing Decreasing Number
- Increment Decrement
- Factorial using recursion
- Fibonacci number
- Sum of digits
- Fibonacci series 2
- Binomial Coefficient
- Tiling Problem
- Pallindrome
- First Occurence
- Last Occurence
- Frequency of element
- Implement Power Function
- Count Binary Strings
24. Object Oriented Programming Introduction
- Introduction to OOPS
- Classes & Objects
- Product Class Demo
- Getters & Setters
- Constructors
- Copy Constructor
- Shallow & Deep Copy
- Copy Assignment Operator
- Destructor
- OOPs
Requirements:
- No Pre-requisites: You don’t need any prior knowledge or experience to enroll in this course.
- Passion to Learn Coding: All you need is a genuine enthusiasm for learning the art of coding.
Who this course is for C++ Programming for Beginners:
- Beginners Eager to Begin Programming: This course is perfect for those who are new to programming and want to take their first steps into the coding world.
- Developers Seeking C++ Syntax Mastery: If you’re a developer aiming to enhance your understanding of C++ syntax, this course will provide you with valuable insights.
- Students Planning to Explore Data Structures & Algorithms in C++: For students with an interest in delving into data structures and algorithms using C++, this course serves as an excellent foundation.
Who Should Take This Course?
Both persons with some coding expertise who want to strengthen their C++ skills and newbies who want to learn C++ programming from start should take this course.
Are There Any Requirements To Take This Course?
There are no requirements needed. By the end of the course, you will have a firm foundation in C++ programming because we start from scratch.
Does This Course Have A Cost?
The course is entirely free. Making high-quality education available to everyone is our goal.
Course Download Links
Download Instructions:
If Download link not loading or working then try with VPN.
Downoad all the parts then extract all zip files into 1 folder.
File Password: nulledsourcecode.com
-
C++ Programming for Beginners (2023) - Video Tutorials - Download Part 1
DISCLAIMER
This course was obtained from a free source and is not hosted on the nulledsourcecode.com website. We can safely say that it is not our responsibility. Use this file whatever you like for your own purposes. Downloading copyrighted material is illegal, and all the files here are only for educational uses. Developers/creator/maker made it with difficulty. We request you to buy a genuine version from it creator/developer/owner's website.